Overview
This project demonstrates how to send data from one board to another using a LoRa (Long Range) communication module. This project needs a transmitter and a receiver. The transmitter waits for user input from the serial monitor. Once input is received it transmits the string via LoRA to the receiver which prints it on its serial monitor.
Components
Both the transmitter and the receiver will use the components listed below.
Component Name | Purpose |
---|---|
Arduino Nano | This will be the microcontroller |
RA02-SX1278 | This will be the LoRa module |
SX1278 antenna | This will be the LoRa module antenna |
Circuit Diagram
Transmitter
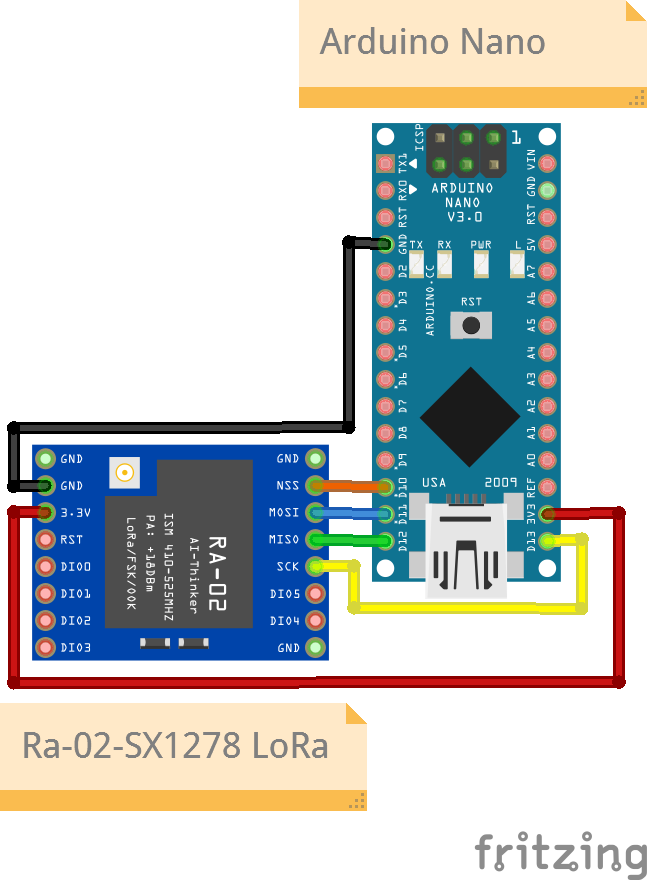
Receiver
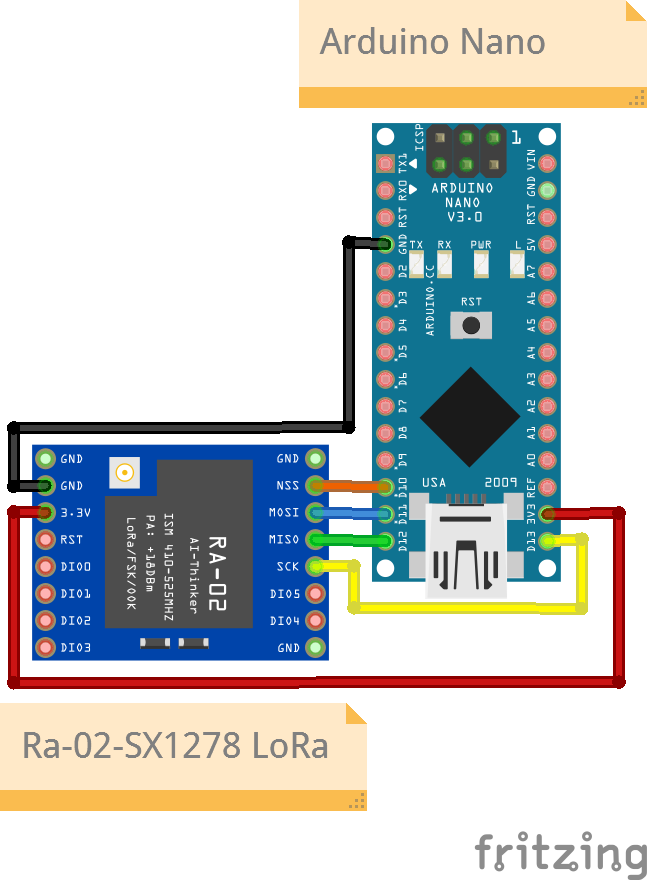
Assembly
Connect the antenna to the SX 1278 Module to improve the range and performance.
RA-02-SX1278 Module
Both the transmitter and the receiver will have the connections listed below.
Arduino Nano Pin | RA-02-SX1278 Pin |
---|---|
D10 | NSS |
D11 | MOSI |
D12 | MISO |
D13 | SCK |
5v | 3v3 |
GND | GND |
Code
Transmitter
/*
Project: LoRa transmitter
Project Description:
This project shows you how to receive data from LoRa and print it serially
Author: STEMVentor Educonsulting
This code is copyrighted. Please do not reuse or share for any purpose other than for learning with subscribed STEMVentor programs.
This code is for educational purposes only and is not for production use.
*/
// LIBRARIES
#include <SPI.h>
#include <LoRa.h>
// PIN DEFINTIONS
//No need to physically connect these pins in this use case but need to be defined for the LoRa to transmit
// Comment if not using Arduino Nano
#define nss 10 //LoRa module NSS pin
#define rst 9 //LoRa module RST pin
#define dio0 2 //LoRa module dio0 pin
// Uncomment if using ESP32
// #define nss 15 //LoRa module NSS pin
// #define rst 16 //LoRa module RST pin
// #define dio0 4 //LoRa module DIO0 pin
// CONSTANT DEFINITIONS
// GLOBAL VARIABLES
// Used for reading a string.
String receivedString;
// LOCAL FUNCTIONS
//setup function for LoRa
void loraSetup()
{
Serial.println("Starting LoRa Communication");
LoRa.setPins(nss, rst, dio0); //These pins need not be connected but have to be defined
if (!LoRa.begin(433E6)) { //starts LoRa at 433MHz frequency
Serial.println("Starting LoRa failed!");
while (1); // runs only once
}
// LoRa.setSyncWord(0xFF); // isolates network , the receiver also needs to have the same syncword. More different the syncwords , better the isolation.
}
//loop function
void sendMessage(String outgoing) //sends the data as a string over LoRa
{
Serial.println("Sending packet id " + outgoing.substring(0,1)) ;
LoRa.beginPacket(); // start packet
LoRa.print(outgoing); // add payload
LoRa.endPacket(); // finish packet and send it
Serial.println("Sent packet id " + outgoing.substring(0,1));
}
//Read an entire string and then transmit it via LoRa (this is a blocking function and waits until timeout).
void readString()
{
Serial.println("Enter a data string to be transmitted via LoRa:");
while (Serial.available() == 0) {} //wait for data available
receivedString = Serial.readString(); //read until timeout
receivedString.trim(); // remove any \r \n whitespace at the end of the String
}
// THE setup FUNCTION RUNS ONCE WHEN YOU PRESS RESET OR POWER THE BOARD.
void setup()
{
// Start the serial communication with baud rate suitable for your components.
Serial.begin(9600);
loraSetup();
Serial.println("The board is ready!");
}
// THE loop FUNCTION RUNS OVER AND OVER AGAIN FOREVER UNTIL THE BOARD IS POWERED OFF.
void loop()
{
readString();
sendMessage(receivedString);
}
Receiver
/*
Project: LoRa receiver
Project Description:
This project shows you how to receive data from LoRa and print it serially
Author: STEMVentor Educonsulting
This code is copyrighted. Please do not reuse or share for any purpose other than for learning with subscribed STEMVentor programs.
This code is for educational purposes only and is not for production use.
*/
// LIBRARIES
#include <SPI.h>
#include <LoRa.h>
// PIN DEFINTIONS
//No need to physically connect these pins in this use case but need to be defined for the LoRa to transmit
// Comment if not using Arduino Nano
#define nss 10 //LoRa module NSS pin
#define rst 9 //LoRa module RST pin
#define dio0 2 //LoRa module dio0 pin
// Uncomment if using ESP32
// #define nss 15 //LoRa module NSS pin
// #define rst 16 //LoRa module RST pin
// #define dio0 4 //LoRa module DIO0 pin
// CONSTANT DEFINITIONS
// GLOBAL VARIABLES
// Used for reading a string.
String receivedString;
// LOCAL FUNCTIONS
//setup function for LoRa
void loraSetup()
{
Serial.println("Starting LoRa Communication");
LoRa.setPins(nss, rst, dio0); //These pins need not be connected but have to be defined
if (!LoRa.begin(433E6)) { //starts LoRa at 433MHz frequency
Serial.println("Starting LoRa failed!");
while (1); // runs only once
}
// LoRa.setSyncWord(0xFF); // isolates network , the receiver also needs to have the same syncword. More different the syncwords , better the isolation.
}
//loop function
void onReceive(int packetSize)
{
if (packetSize == 0) return; // if there's no packet, return
receivedString = "";
while (LoRa.available()) {
receivedString += (char)LoRa.read();
}
Serial.println(receivedString);
}
// THE setup FUNCTION RUNS ONCE WHEN YOU PRESS RESET OR POWER THE BOARD.
void setup()
{
// Start the serial communication with baud rate suitable for your components.
Serial.begin(9600);
loraSetup();
Serial.println("The board is ready!");
}
// THE loop FUNCTION RUNS OVER AND OVER AGAIN FOREVER UNTIL THE BOARD IS POWERED OFF.
void loop()
{
onReceive(LoRa.parsePacket()); //constantly listen for incoming data
}