Overview
This project demonstrates how to use a switch to control the state of an external LED connected to a microcontroller. There are two kinds of switches:
- On/Off Switch: The switch has two positions on and off and remains in either position unless moved to the other position (it does not have to be held in a position).
- Pushbutton Switch: When the pushbutton is pressed, the LED turns on and remains on as long as the pushbutton remains pressed; when released, it turns off.
In this project we will use the on/off switch, in the next we will use the pushbutton switch.
Components
Component Name | Purpose |
---|---|
Arduino Nano | The microcontroller. |
4-pin Self-Lock Switch | The on/off switch. |
2-pin LED | The LED controlled by the switches. |
Circuit Diagram
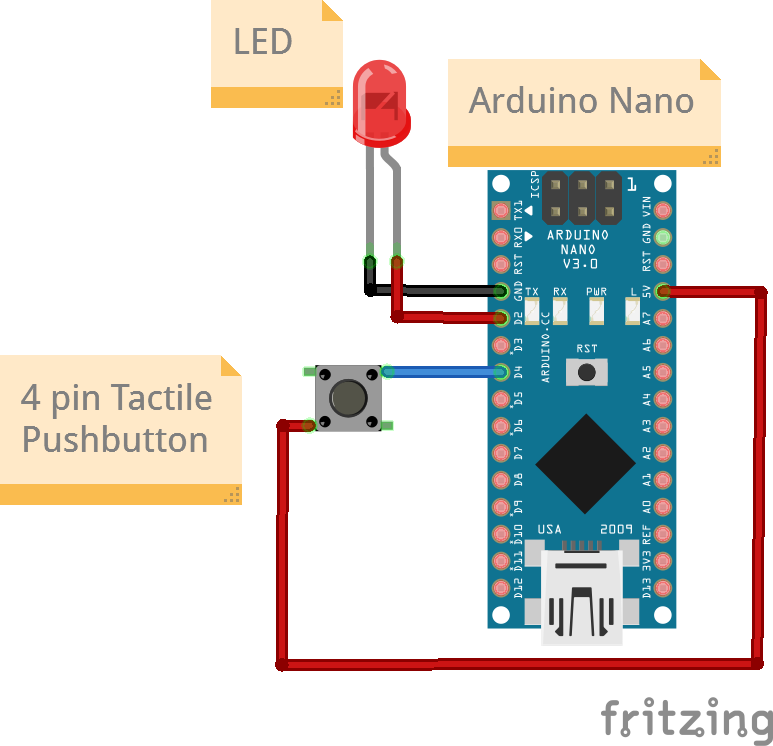
Assembly
4-pin Self-Lock Switch
Arduino Nano Pin | Switch Pin |
---|---|
D12 | Pin1 |
5v | Pin2 |
2-pin LED
Arduino Nano Pin | LED Pin |
---|---|
D2 | Anode (longer leg) |
GND | Cathode (shorter leg) |
Code
/*
Project: Pushbutton controlling an external LED.
Project Description:
This project will show you how to read a digital input from a pushbutton and send a digital signal to a pin to turn an external LED on or off.
Author: STEMVentor Educonsulting
This code is copyrighted. Please do not reuse or share for any purpose other than for learning with subscribed STEMVentor programs.
This code is for educational purposes only and is not for production use.
*/
// LIBRARIES
// PIN DEFINITIONS
// In a 4-pin push button the two pins on the same long side are connected together.
const int pushbuttonPin = 4; // The pushbutton pin
// In an LED the longer pin is the anode (+ve) and is connected to the signal pin.
// The shorter pin is the cathode (-ve) and is connected to ground.
const int ledPin = 2; // The LED pin
// CONSTANT DEFINITIONS
// GLOBAL VARIABLES
bool buttonValue = false; // For reading the pushbutton status.
// LOCAL FUNCTIONS
void buttonSetup()
{
// initialize the pushbutton pin as an input.
pinMode(pushbuttonPin, INPUT);
}
void ledSetup()
{
// Initialize the LED pin as an output.
pinMode(ledPin, OUTPUT);
digitalWrite(ledPin, LOW);
}
void readButtonValue()
{
// Read the value of the pushbutton state.
buttonValue = digitalRead(pushbuttonPin);
}
void digitalWriteLED(bool value)
{
// If the button is pressed the buttonValue is HIGH.
if (value == true) {
Serial.println("LED HIGH");
// Turn LED on.
digitalWrite(ledPin, HIGH);
} else {
// Turn LED off.
Serial.println("LED LOW");
digitalWrite(ledPin, LOW);
}
}
// THE setup FUNCTION RUNS ONCE WHEN YOU PRESS RESET OR POWER THE BOARD.
void setup() {
Serial.begin(9600);
buttonSetup();
ledSetup();
Serial.println("The board is ready!");
}
// THE loop FUNCTION RUNS OVER AND OVER AGAIN FOREVER UNTIL THE BOARD IS POWERED OFF.
void loop() {
readButtonValue();
digitalWriteLED(buttonValue);
}